Well, apparently your pads send the same MIDI message no matter what mode you are on so I created a sample file that might help you grasp the concept of what to do.
In this example the first translator (0.1) takes note 64 (0x41) On MIDI Channel 1 as input. In the rules, we increment the global variable “ga” so that it cycles from 0-7. We will later use this variable to change the outgoing MIDI channel for the pads so that they will be unique when sending to Rekordbox.
Here are the rules. I added a log message so that you can see the current output channel in the log window. We do not send any output on this translator.
// Set MIDI channel
// here we go between 0 and 7
// for mode 1-8
ga=ga+1
if ga==8 then ga=0
Log "Log Mode is %ga%"
Then in translator 0.1 we process pad messages. I takes Any note on MIDI CH 6 for input, however the rules will only process messages for 20-27 (0x14-0x1B).
if pp<20 then exit rules, skip Outgoing Action
if pp>27 then exit rules, skip Outgoing Action
On output we send the same note number as input but on a different MIDI channel as specified by the current value of “ga”.
Here is a log of the output:
10594151 - 1.0:6 Log Mode is 1
10594153 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=20 and velocity:127 (0x7F)
10594153 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=21 and velocity:127 (0x7F)
10594153 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=21 and velocity:127 (0x7F)
10594153 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=22 and velocity:127 (0x7F)
10594153 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=23 and velocity:127 (0x7F)
10594209 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=24 and velocity:127 (0x7F)
10594209 - OUT 1.1 Note On on ch.'ga'=1 (ch.2) with note:pp=25 and velocity:127 (0x7F)
10594890 - 1.0:6 Log Mode is 2
10594891 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=20 and velocity:127 (0x7F)
10594891 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=21 and velocity:127 (0x7F)
10594891 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=21 and velocity:127 (0x7F)
10594891 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=22 and velocity:127 (0x7F)
10594891 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=23 and velocity:127 (0x7F)
10594957 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=24 and velocity:127 (0x7F)
10594957 - OUT 1.1 Note On on ch.'ga'=2 (ch.3) with note:pp=25 and velocity:127 (0x7F)
10595548 - 1.0:6 Log Mode is 3
10595552 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=20 and velocity:127 (0x7F)
10595552 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=21 and velocity:127 (0x7F)
10595552 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=21 and velocity:127 (0x7F)
10595552 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=22 and velocity:127 (0x7F)
10595552 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=23 and velocity:127 (0x7F)
10595607 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=24 and velocity:127 (0x7F)
10595607 - OUT 1.1 Note On on ch.'ga'=3 (ch.4) with note:pp=25 and velocity:127 (0x7F)
10596259 - 1.0:6 Log Mode is 4
10596267 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=20 and velocity:127 (0x7F)
10596267 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=21 and velocity:127 (0x7F)
10596267 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=21 and velocity:127 (0x7F)
10596267 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=22 and velocity:127 (0x7F)
10596267 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=23 and velocity:127 (0x7F)
10596267 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=24 and velocity:127 (0x7F)
10596333 - OUT 1.1 Note On on ch.'ga'=4 (ch.5) with note:pp=25 and velocity:127 (0x7F)
10597024 - 1.0:6 Log Mode is 5
10597026 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=20 and velocity:127 (0x7F)
10597026 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=21 and velocity:127 (0x7F)
10597026 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=21 and velocity:127 (0x7F)
10597026 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=22 and velocity:127 (0x7F)
10597026 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=23 and velocity:127 (0x7F)
10597093 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=24 and velocity:127 (0x7F)
10597093 - OUT 1.1 Note On on ch.'ga'=5 (ch.6) with note:pp=25 and velocity:127 (0x7F)
10597975 - 1.0:6 Log Mode is 6
10597978 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=20 and velocity:127 (0x7F)
10597979 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=21 and velocity:127 (0x7F)
10597979 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=21 and velocity:127 (0x7F)
10597979 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=22 and velocity:127 (0x7F)
10597979 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=23 and velocity:127 (0x7F)
10598034 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=24 and velocity:127 (0x7F)
10598034 - OUT 1.1 Note On on ch.'ga'=6 (ch.7) with note:pp=25 and velocity:127 (0x7F)
10598666 - 1.0:6 Log Mode is 7
10598674 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=20 and velocity:127 (0x7F)
10598674 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=21 and velocity:127 (0x7F)
10598674 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=21 and velocity:127 (0x7F)
10598674 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=22 and velocity:127 (0x7F)
10598674 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=23 and velocity:127 (0x7F)
10598674 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=24 and velocity:127 (0x7F)
10598740 - OUT 1.1 Note On on ch.'ga'=7 (ch.8) with note:pp=25 and velocity:127 (0x7F)
I also added a MIDI thru path that is not covered for any translators. I have all translators set with the option of swallow so those incoming notes will not go through the MIDI thru path.
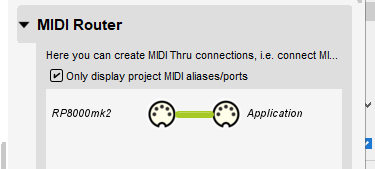
Finally I set the preset level input and output to your controller and application respectively. This will prevent notes coming from any other source to trigger the translators and ensure the translator output is directed to the proper destination port.
Here is the project file:
Channel-Rotation-Example-2023-01-18.bmtp (2.0 KB)
Steve Caldwell
Bome Customer Care
Also available for paid consulting services: bome@sniz.biz